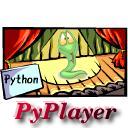
|
PyPlayer: Python Client for Player/Stage
Function |
get_position()
|
---|
Parameters |
index=0
|
---|
Return |
tuple of an odometry tuple, a speed tuple, and a stall flag.
The odometry tuple is (x-position, y-position, heading), and
the speed tuple is (x-speed, y-speed, turnrate). The stall flag
is 1 if a motor get stalled, and is 0 otherwise.
|
---|
Description |
Return the latest data of a position device.
|
---|
Example |
>>> robot.get_position()
((291, 244, 38), (100, 0, 20), 0)
>>>
|
---|
Function |
set_position()
|
---|
Parameters |
xpos=None, ypos=None, yawpos=None, xspeed=None, yspeed=None, yawspeed=None,
index=0
|
---|
Return |
None
|
---|
Description |
Change the position and the speed of a robot. If a parameter is None,
the current value will be used; therefore, the value is not supposed
to change theoretically. However, the value may change because of the
communication delay.
|
---|
Example |
>>> robot.set_position(2000, 1000, 90)
>>>
|
---|
Function |
set_speed()
|
---|
Parameters |
xspeed=None, yspeed=None, yawspeed=None, xpos=None, ypos=None, yawpos=None,
index=0
|
---|
Return |
None
|
---|
Description |
Change the speed and the position of a robot. If a parameter is None,
the current value will be used; therefore, the value is not supposed
to change theoretically. However, the value may change because of the
communication delay.
|
---|
Example |
>>> robot.set_speed(300, 0, 45)
>>>
|
---|
Function |
get_position_geometry()
|
---|
Parameters |
None
|
---|
Return |
tuple of a pose tuple and a size tuple. The pose tuple is (x-position,
y-position, heading), and the size tuple is (width, height).
|
---|
Description |
Request robot geometry (pose and size).
|
---|
Example |
>>> robot.get_position_geometry()
((65496, 0, 0), (440, 330))
>>>
|
---|
Function |
turnon_motors()
|
---|
Parameters |
None
|
---|
Return |
None
|
---|
Description |
Turn on the motor power of a robot.
|
---|
Example |
>>> robot.turnon_motors()
>>>
|
---|
Function |
turnoff_motors()
|
---|
Parameters |
None
|
---|
Return |
None
|
---|
Description |
Turn off the motor power of a robot.
|
---|
Example |
>>> robot.turnoff_motors()
>>>
|
---|
Function |
set_position_control()
|
---|
Parameters |
mode='velocity'
|
---|
Return |
None
|
---|
Description |
Set position control mode. There are two different modes:
- 'velocity' : receive velocity commands
- 'position' : receive position commands
|
---|
Example |
>>> robot.set_position_control('position')
>>>
|
---|
Function |
set_velocity_control()
|
---|
Parameters |
mode='default'
|
---|
Return |
None
|
---|
Description |
Set velocity control mode. there are four different modes:
- 'default' : take the system default mode. ('separate' for
P2OS and 'pd' for REB)
- 'direct' : direct wheel control
- 'separate' : separate translational and rotational control
- 'pd' : velocity-based heading PD control
|
---|
Example |
>>> robot.set_velocity_mode('direct')
>>>
|
---|
Function |
reset_odometry()
|
---|
Parameters |
None
|
---|
Return |
None
|
---|
Description |
Reset odometry information to (0,0,0).
|
---|
Example |
>>> robot.reset_odometry()
>>>
|
---|
Function |
set_odometry()
|
---|
Parameters |
x, y, theta
|
---|
Return |
None
|
---|
Description |
Set odometry information to (x,y,theta).
|
---|
Example |
>>> robot.reset_odometry()
>>>
|
---|
Function |
set_pid_velocity()
|
---|
Parameters |
kp, ki, kd
|
---|
Return |
None
|
---|
Description |
Set the parameters of a PID controller for velocity control.
|
---|
Example |
>>> robot.set_pid_velocity(0.3 0.001, 0.05)
>>>
|
---|
Function |
set_pid_position()
|
---|
Parameters |
kp, ki, kd
|
---|
Return |
None
|
---|
Description |
Set the parameters of a PID controller for position control.
|
---|
Example |
>>> robot.set_pid_velocity(0.3 0.001, 0.05)
>>>
|
---|
Function |
set_speed_profile()
|
---|
Parameters |
max_speed, max_acc
|
---|
Return |
None
|
---|
Description |
Set the maximum speed and the maximum acceleration.
|
---|
Example |
>>> robot.set_speed_profile(1000, 200)
>>>
|
---|
|
|
|