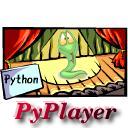
|
PyPlayer: Python Client for Player/Stage
Function |
get_gripper()
|
---|
Parameters |
index=0
|
---|
Return |
tuple of a status tuple and a beams tuple.
The status tuple is (paddles_open, paddes_closed, paddles_moving,
paddles_error, lift_up, lift_down, lift_moving, lift_error), and
the beams tuple (gripper_limit_reached, lift_limit_reached,
outer_beam_obstructed, inner_beam_obstructed, left_paddle_open,
right_paddle_open).
'1' indicates 'yes' and '0' indicates 'no'.
|
---|
Description |
Return the latest data of a gripper device.
|
---|
Example |
>>> robot.get_gripper()
((1, 0, 0, 0, 0, 1, 0, 0), (0, 0, 0, 0, 0, 0))
>>>
|
---|
Function |
gripper_open()
|
---|
Parameters |
index=0
|
---|
Return |
None
|
---|
Description |
Send the 'open' command to a gripper.
|
---|
Example |
>>> robot.gripper_open()
>>>
|
---|
Function |
gripper_close()
|
---|
Parameters |
index=0
|
---|
Return |
None
|
---|
Description |
Send the 'close' command to a gripper.
|
---|
Example |
>>> robot.gripper_close()
>>>
|
---|
Function |
gripper_stop()
|
---|
Parameters |
index=0
|
---|
Return |
None
|
---|
Description |
Send the 'stop' command to a gripper.
|
---|
Example |
>>> robot.gripper_stop()
>>>
|
---|
Function |
gripper_up()
|
---|
Parameters |
index=0
|
---|
Return |
None
|
---|
Description |
Send the 'up' command to a gripper.
|
---|
Example |
>>> robot.gripper_up()
>>>
|
---|
Function |
gripper_down()
|
---|
Parameters |
index=0
|
---|
Return |
None
|
---|
Description |
Send the 'down' command to a gripper.
|
---|
Example |
>>> robot.gripper_down()
>>>
|
---|
Function |
gripper_stay()
|
---|
Parameters |
index=0
|
---|
Return |
None
|
---|
Description |
Send the 'stay' command to a gripper.
|
---|
Example |
>>> robot.gripper_stay()
>>>
|
---|
Function |
gripper_store()
|
---|
Parameters |
index=0
|
---|
Return |
None
|
---|
Description |
Send the 'store' command to a gripper.
|
---|
Example |
>>> robot.gripper_store()
>>>
|
---|
Function |
gripper_deploy()
|
---|
Parameters |
index=0
|
---|
Return |
None
|
---|
Description |
Send the 'deploy' command to a gripper.
|
---|
Example |
>>> robot.gripper_deploy()
>>>
|
---|
Function |
gripper_halt()
|
---|
Parameters |
index=0
|
---|
Return |
None
|
---|
Description |
Send the 'halt' command to a gripper.
|
---|
Example |
>>> robot.gripper_halt()
>>>
|
---|
Function |
gripper_press()
|
---|
Parameters |
press_time=1, index=0
|
---|
Return |
None
|
---|
Description |
Send the 'press' command to a gripper.
|
---|
Example |
>>> robot.gripper_press()
>>>
|
---|
Function |
gripper_carry()
|
---|
Parameters |
carry_time=1, index=0
|
---|
Return |
None
|
---|
Description |
Send the 'carry' command to a gripper.
|
---|
Example |
>>> robot.gripper_carry()
>>>
|
---|
Function |
is_paddles_open()
|
---|
Parameters |
index=0
|
---|
Return |
the current status (1/0)
|
---|
Description |
Check if paddels are open.
|
---|
Example |
>>> robot.is_paddles_open()
1
>>>
|
---|
Function |
is_paddles_closed()
|
---|
Parameters |
index=0
|
---|
Return |
the current status (1/0)
|
---|
Description |
Check if paddels are closed.
|
---|
Example |
>>> robot.is_paddles_closed()
0
>>>
|
---|
Function |
is_paddles_moving()
|
---|
Parameters |
index=0
|
---|
Return |
the current status (1/0)
|
---|
Description |
Check if paddels are moving.
|
---|
Example |
>>> robot.is_paddles_moving()
0
>>>
|
---|
Function |
is_paddles_error()
|
---|
Parameters |
index=0
|
---|
Return |
the current status (1/0)
|
---|
Description |
Check if there is an error related to paddles.
|
---|
Example |
>>> robot.is_paddles_error()
0
>>>
|
---|
Function |
is_lift_up()
|
---|
Parameters |
index=0
|
---|
Return |
the current status (1/0)
|
---|
Description |
Check if a lift is in up position.
|
---|
Example |
>>> robot.is_lift_up()
0
>>>
|
---|
Function |
is_lift_down()
|
---|
Parameters |
index=0
|
---|
Return |
the current status (1/0)
|
---|
Description |
Check if a lift is in down position.
|
---|
Example |
>>> robot.is_lift_down()
1
>>>
|
---|
Function |
is_lift_moving()
|
---|
Parameters |
index=0
|
---|
Return |
the current status (1/0)
|
---|
Description |
Check if a lift is moving.
|
---|
Example |
>>> robot.is_lift_moving()
0
>>>
|
---|
Function |
is_lift_error()
|
---|
Parameters |
index=0
|
---|
Return |
the current status (1/0)
|
---|
Description |
Check if there is an error related to a lift.
|
---|
Example |
>>> robot.is_lift_error()
0
>>>
|
---|
Function |
is_glimit_reached()
|
---|
Parameters |
index=0
|
---|
Return |
the current status (1/0)
|
---|
Description |
Check if a gripper is reached its limit.
|
---|
Example |
>>> robot.is_glimit_reached()
0
>>>
|
---|
Function |
is_llimit_reached()
|
---|
Parameters |
index=0
|
---|
Return |
the current status (1/0)
|
---|
Description |
Check if a lift is reached its limit.
|
---|
Example |
>>> robot.is_llimit_reached()
0
>>>
|
---|
Function |
is_obeam_obstructed()
|
---|
Parameters |
index=0
|
---|
Return |
the current status (1/0)
|
---|
Description |
Check if an outer beam is obstructed.
|
---|
Example |
>>> robot.is_obeam_obstructed()
0
>>>
|
---|
Function |
is_ibeam_obstructed()
|
---|
Parameters |
index=0
|
---|
Return |
the current status (1/0)
|
---|
Description |
Check if an inner beam is obstructed.
|
---|
Example |
>>> robot.is_ibeam_obstructed()
1
>>>
|
---|
Function |
is_lpaddle_open()
|
---|
Parameters |
index=0
|
---|
Return |
the current status (1/0)
|
---|
Description |
Check if a left paddle is open.
|
---|
Example |
>>> robot.is_lpaddle_open()
0
>>>
|
---|
Function |
is_rpaddle_open()
|
---|
Parameters |
index=0
|
---|
Return |
the current status (1/0)
|
---|
Description |
Check if a right paddle is open.
|
---|
Example |
>>> robot.is_rpaddle_open()
0
>>>
|
---|
|
|
|